Machine learning is revolutionizing the tech world, and Python is at the forefront of this transformation. If you’re a Python developer looking to dive into machine learning, this step-by-step guide will walk you through building your first machine learning project in Python. By the end of this tutorial, you’ll have a solid foundation to integrate machine learning into applications.
Introduction
In today’s tech-driven world, understanding machine learning (ML) can significantly elevate your development skills. Python, with its robust libraries and simplicity, is the go-to language for ML. This guide will show you how to build your first machine learning project with Python, breaking down the process into manageable steps. Whether you’re a beginner or looking to refresh your skills, this tutorial aims to provide easy-to-follow and highly informative guidance.
Understanding Machine Learning
Before we dive into coding, it’s essential to understand what machine learning is. Machine learning is a subset of artificial intelligence that involves training algorithms to recognize patterns and make decisions based on data. There are various types of machine learning, including supervised, unsupervised, and reinforcement learning. In this guide, we’ll focus on supervised learning, which is the most common starting point for beginners.
Setting Up Your Environment
First, ensure you have Python installed on your system. You can download it from the official Python website. Next, you’ll need to install several essential libraries: NumPy, Pandas, Matplotlib, and Scikit-Learn. You can install these using pip:
pip install numpy pandas matplotlib scikit-learn
Step 1: Importing Libraries
Let’s start by importing the necessary libraries. Open your preferred Python IDE or a Jupyter notebook and run the following code:
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
Step 2: Loading the Dataset
For this tutorial, we’ll use a simple dataset – the California Housing dataset, which is available in Scikit-Learn. This dataset contains information about various houses in California, including the median value of owner-occupied homes.
from sklearn.datasets import fetch_california_housing
housing = fetch_california_housing()
data = pd.DataFrame(housing.data, columns=housing.feature_names)
data['PRICE'] = housing.target
Step 3: Exploring the Data
Understanding your data is crucial. Let’s look at the first few rows and some basic statistics.
print(data.head())
print(data.describe())
Step 4: Preprocessing the Data for Machine Learning
Data preprocessing is a critical step in machine learning. It involves cleaning and transforming data to prepare it for modeling. For simplicity, we’ll handle missing values and split the data into training and testing sets.
# Check for missing values
print(data.isnull().sum())
# Split the data
X = data.drop('PRICE', axis=1)
y = data['PRICE']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Step 5: Building the Machine Learning Model
Now, it’s time to build our model. We’ll use a simple linear regression model for this tutorial.
model = LinearRegression()
model.fit(X_train, y_train)
Step 6: Evaluating the Machine Learning Model
Evaluating your model helps you understand how well it performs on unseen data. We’ll use mean squared error (MSE) as our evaluation metric.
y_pred = model.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
print(f'Mean Squared Error: {mse}')
Step 7: Visualizing the Results
Visualization helps in interpreting the results effectively. Let’s plot the predicted vs. actual values.
plt.scatter(y_test, y_pred)
plt.xlabel('Actual Prices')
plt.ylabel('Predicted Prices')
plt.title('Actual vs Predicted Prices')
plt.show()
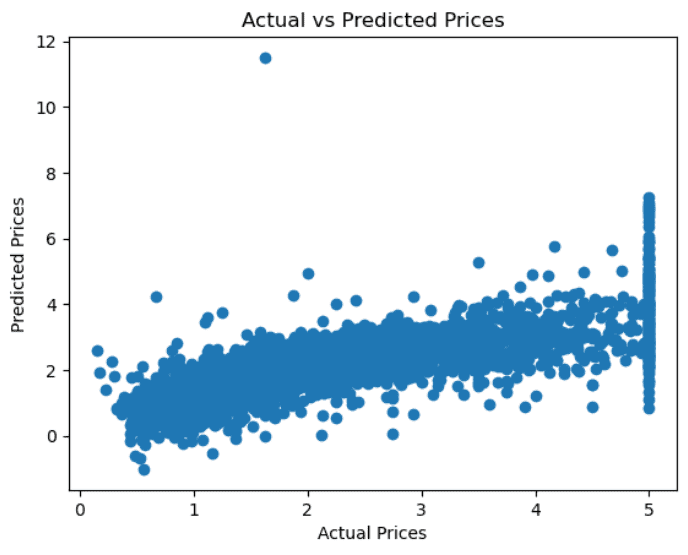
This is the scatterplot of Actual Prices vs Predicted Prices from our model. Note there is an outlier that needs to be investigated.
Step 8: Improving the Machine Learning Model
Improving a model involves experimenting with different algorithms, tuning hyperparameters, and feature engineering. Here are some suggestions to improve your model:
- Feature Engineering: Create new features that might help the model learn better.
- Hyperparameter Tuning: Depending on the algorithm used, you could use techniques like GridSearchCV to find the best parameters for your model.
- Try Different Algorithms: Experiment with other algorithms like K-Nearest Neighbours, Logistic Regression, Decision Trees, Random Forests or Gradient Boosted Machines
Resources
If you wish to learn more about machine learning, there are plenty of courses available on Udemy. And there are plenty of videos available on YouTube. Contact me if you wish to find out more. Keep an eye out for other articles here.
Leave a Reply